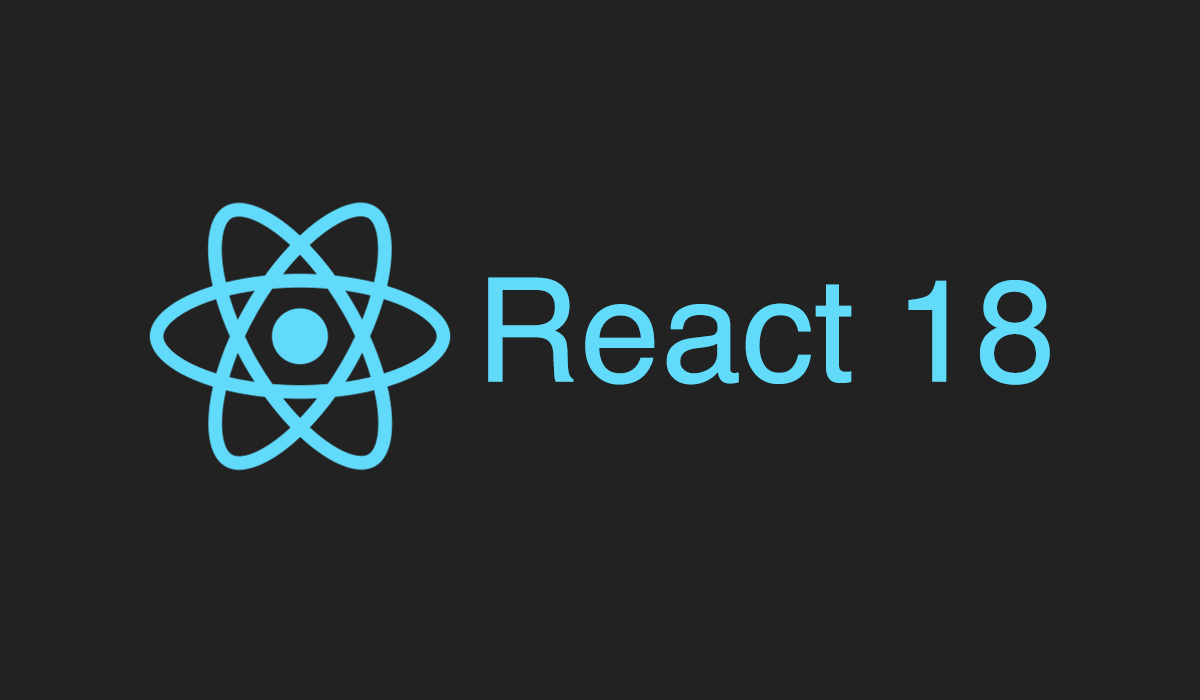
React 18 - Here's What's New
React 18 is here! The latest release, including optimizations and a few new features, is now available on NPM. Here’s what’s new…
React 18 is here! The latest release, including optimizations and a few new features, is now available on NPM. Here’s what’s new…
The following pattern is fairly common in other technologies:
This won’t work in salesforce. By rethrowing the exception (assuming it’s
not caught further up the call stack), the database transaction will
be rolled back - that includes whatever insert
or update
ws triggered in
logException(e)
. So how do we ensure that the logging occurs in a separate
database transaction? The answer is a platform event!
Conditional rendering in React is pretty straightforward and we do it everywhere. In some cases, though, a tiny abstraction can clean up our code and keep us from repeating ourselves. Here’s a simple Higher Order Component (HOC) for conditionally rendering a component or fragment if-and-only-if it has children.
Apex leaves much to be desired when it comes to mocking callouts to external services. Use of the HttpCalloutMock interface requires that a test author configure mock responses for ALL callouts made through the course of a test’s processing, and this can be quite unmanageable in large or complex projects. There must be a better way… Enter the HTTP Mock Registry.
Frustrated with messy tests and abstraction leaks, we’ve devised a utility that provides a intuitive interface for mocking callouts declaratively as well as a foundation for more modular test suites whenever callouts are involved.
The ability to mock out components is essential to writing quality tests, especially when you’re dealing with integrations or external API invocation. And let’s face it - the out-of-the-box Stub API provided in Apex is just plain awful. Here’s a lightweight utility I threw together to simplify mocking.